Using Zustand with Redux Devtools
Zustand is pretty amazing. It has quickly become the most popular store manager for React and Next.js applications. However, you probably met Redux and Redux Devtools since they were probably the most popular tools for state management before Zustand appears.
If so, you probably know that Redux Devtools are really intuitive and offers a great developer experience, and that's why today we are goint to use Zustand with Redux Devtools.
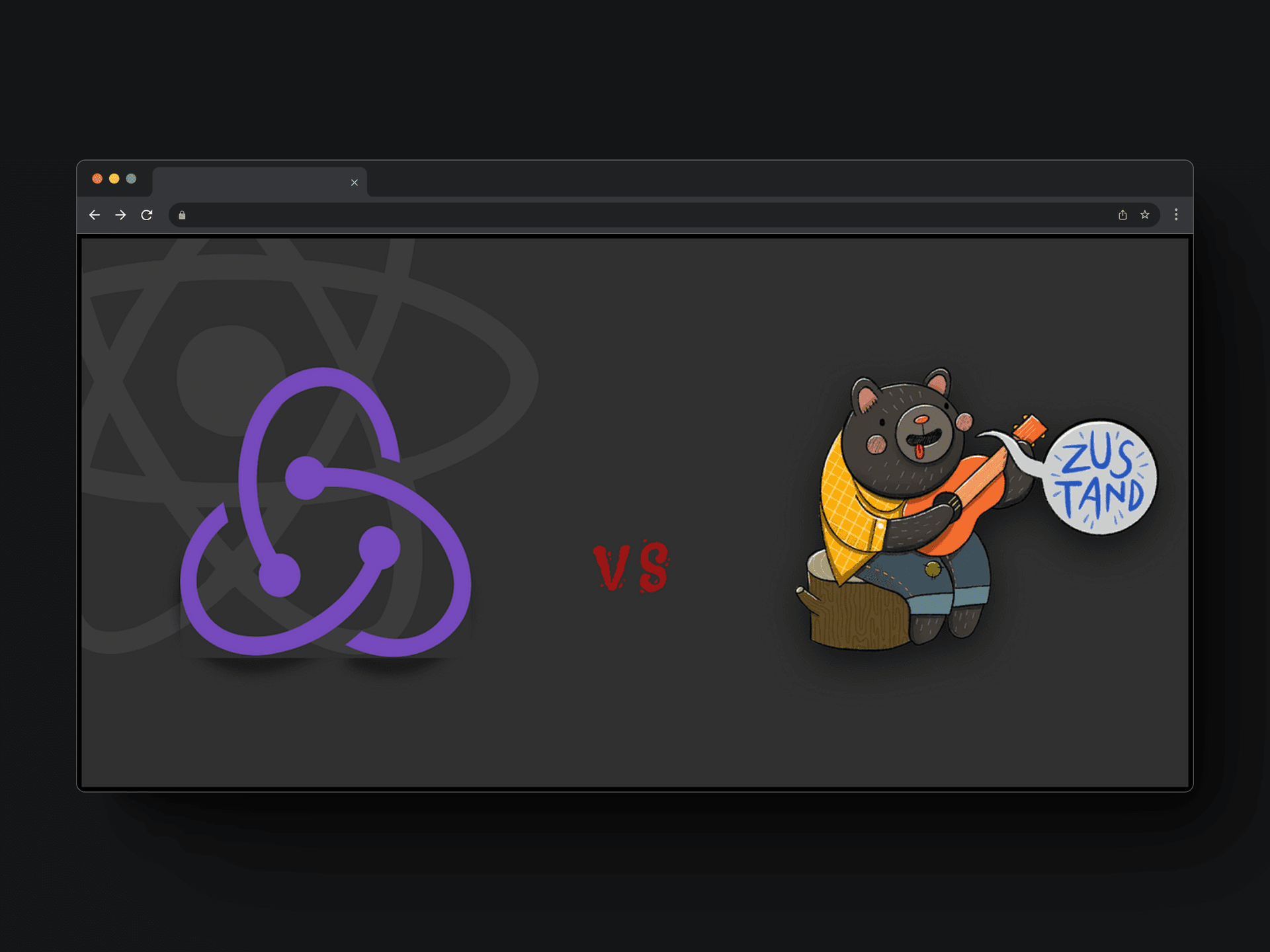
Written by Gabriel Maestre.
Installing dependencies
Once we have set up our React or Next.js application, let's install Zustand.
npm install zustand
Setting up our Store
Once Zustand is installed, let's create a file at the root of our directory called store/. Now let's define our store file. For this example I will create a file called store.js.
import { create } from 'zustand'
const useStore = create((set) => ({
count: 1,
inc: () => set((state) => ({ count: state.count + 1 })),
}))
In case you're wondering, of course, you can always use Typescript instead of Javascript, which is actually recommended, but for this example I'm going to be using Javascript. You may check Zustand documentation to get more information and an example.
Let's prepare for Redux Devtools
Setting up the Redux Devtools with Zustand is a peace of cake and can be done in two steps. First, in our file, we are goint to import a Middleware called devtools from 'zustand/middleware'.
import { create } from 'zustand'
import { devtools } from "zustand/middleware";
const useStore = create((set) => ({
count: 1,
inc: () => set((state) => ({ count: state.count + 1 })),
}))
Now comes the fun part: we need to wrap our store with that middleware.
import { create } from 'zustand'
import { devtools } from "zustand/middleware";
const useStore = create((devtools(set) => ({
count: 1,
inc: () => set((state) => ({ count: state.count + 1 })),
})))
All set up! We can now refresh our page and enjoy our Zustand Store with the Redux Devtools.
Conclusion
Zustand is an amazing state management tool for React and Next.js applications, offering simplicity and powerful features. Integrating it with Redux Devtools enhances the developer experience by providing intuitive debugging and state tracking.
With the given steps, you can leverage the benefits of both Zustand and Redux Devtools, making state management in your applications smoother and more efficient.